Azure Functions for the Enterprise - Exposing Endpoints through Functions
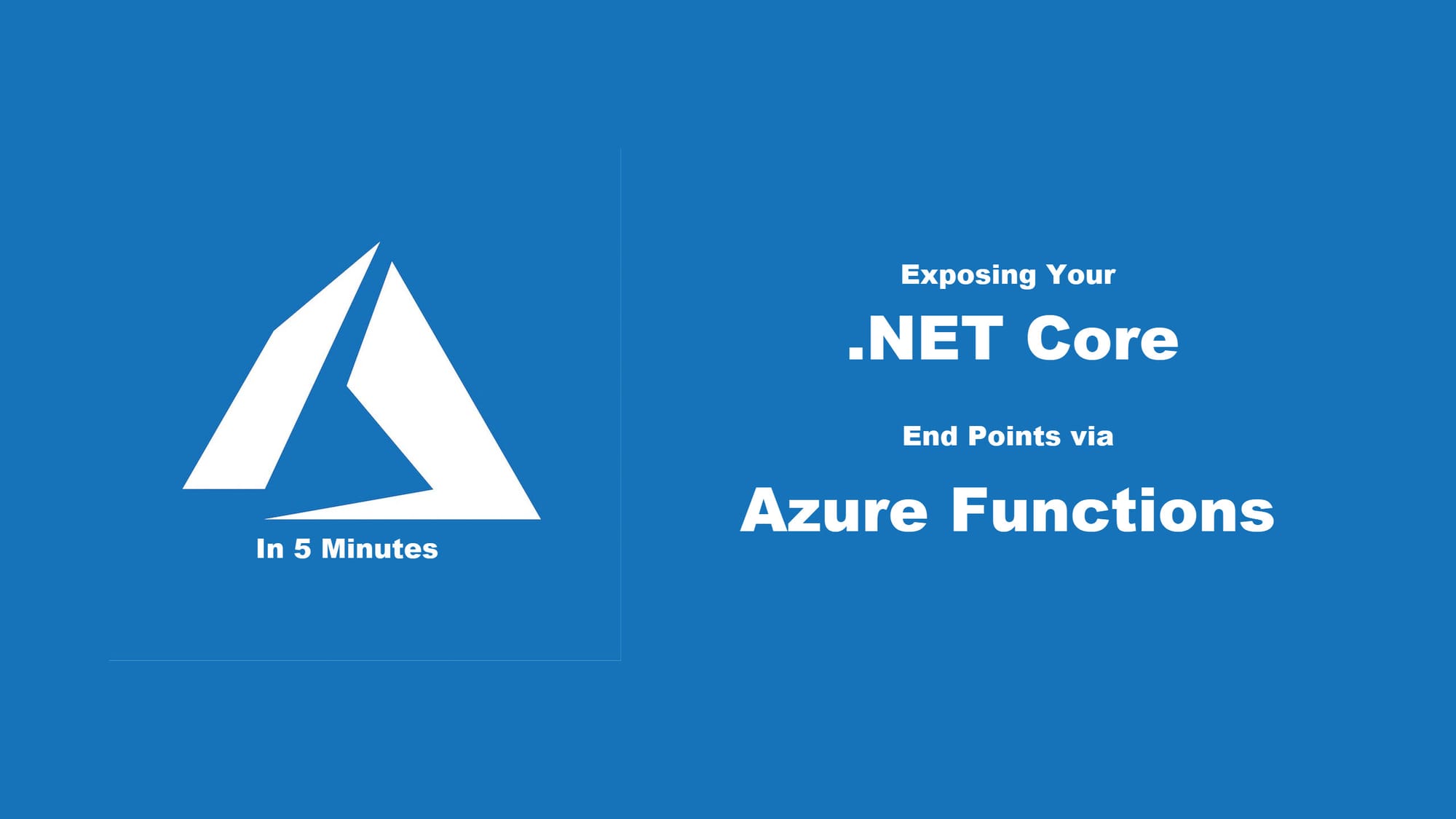
In a previous post, I shared a concept I've been working on to replace different areas of an enterprise application with Azure Functions, but still keep all the best practices. In this post I'm going to cover a very practical way to expose the endpoints of your .NET Core application, usually exposed via an API or MVC layer, through Azure Functions.
The source code covered in this series is in this GitHub repository.
If you find it easier, you can follow the steps in the video below.
Here are the steps we're going to follow:
Prerequisites
- Install Visual Studio 2017 and ensure that the Azure development workload is also installed.
- Make sure you have the latest Azure Functions tools.
- If you don't have an Azure subscription, create a free account before you begin.
1. Create a new Azure Functions project in Visual Studio
Once we have all the prerequisites sorted, we can create a new Azure Functions project in Visual Studio following File > New > Project.
We have a few options here, in my case I'm using .NET Core, but you can still go for .NET Framework. The selection in #2 won't make that much different in a long term, that's just the initial function you are creating, although depending on what you select, you will need an storage account.
2. Reference application layer and configure dependency injection
I've got a whole post about configuring dependency injection within Azure Functions and at the time, the nuget package I found only supported .NET Framework, they are supporting .NET Standard now which should apply nicely for your .NET Core implementation. In any case, here's how my DiConfig
looks:
public class DiConfig
{
public DiConfig()
{
DependencyInjection.Initialize(builder =>
{
builder
.RegisterAssemblyTypes(typeof(GiveMeSomeValuesQuery).Assembly)
.AsImplementedInterfaces();
});
}
}
3. Update your function to inject your query/command
Now you can just inject your query into the function and be happy. In my case, it looks something around these lines.
//here's my DI configuration
[DependencyInjectionConfig(typeof(DiConfig))]
public static class Function1
{
[FunctionName("Function1")]
public static IActionResult Run(
[HttpTrigger(AuthorizationLevel.Function, "get", "post", Route = null)]HttpRequest req,
TraceWriter log,
//here's where I'm injecting my query
[Inject] IGiveMeSomeValuesQuery query)
{
return new OkObjectResult(query.Execute(SortOrder.Ascending));
}
}
4. What next? Right-click > Publish
Well, I know Friends don't let friends right-click publish, but for this example, it really doesn't hurt, we'll configure the CI/CD pipeline in a later post.
Cheers!