Getting Started With SignalR, .NET Core 2.1 And Angular
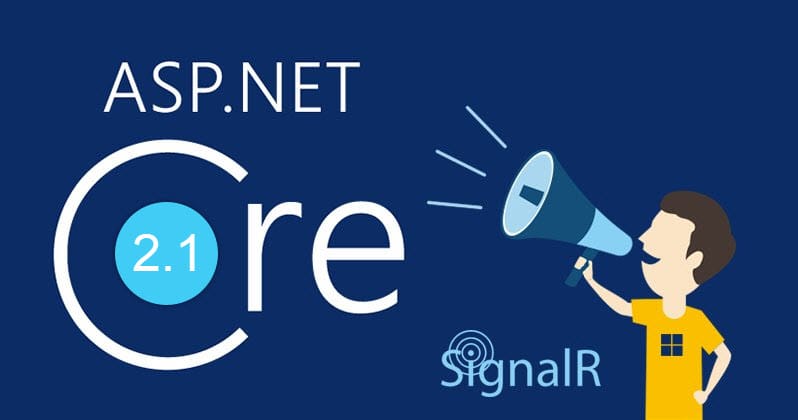
About 3 months ago I wrote a post with the steps to set up SignalR for a new .NET Core 2.0 app with Angular. I thought it was really amazing how easy it was, but now that .NET Core 2.1 is available, no messing up with non-default npm and nuget sources required. And also SignalR comes by default.
Make sure you have the latest .NET Core SDK installed
Getting started
dotnet new angular
cd ClientApp
npm install @aspnet/signalr
Creating new hub in the server side
This is like a controller in a MVC application which is responsible for interactions between the client and the server.
...
using Microsoft.AspNetCore.SignalR;
namespace SignalRDotNetCore21.Hubs
{
public class EchoHub : Hub
{
//you're going to invoke this method from the client app
public void Echo(string message)
{
//you're going to configure your client app to listen for this
Clients.All.SendAsync("Send", message);
}
}
}
Wiring up the server side in the Startup
In the startup.cs
there are really only two things to put together.
public void ConfigureServices(IServiceCollection services)
{
...
services.AddSignalR();
}
public void Configure(IApplicationBuilder app, IHostingEnvironment env)
{
...
// If you're using the SPA template, this should come before app.UseSpa(...);
app.UseSignalR(routes =>
{
routes.MapHub<EchoHub>("/hubs/echo");
});
}
Wiring up client side
For testing purposes, I'm configuring the connection straight into the home component.
import { Component, OnInit } from '@angular/core';
import { HubConnection, HubConnectionBuilder } from '@aspnet/signalr';
@Component({
selector: 'app-home',
templateUrl: './home.component.html',
})
export class HomeComponent implements OnInit {
public hubConnection: HubConnection;
public messages: string[] = [];
public message: string;
ngOnInit() {
let builder = new HubConnectionBuilder();
// as per setup in the startup.cs
this.hubConnection = builder.withUrl('/hubs/echo').build();
// message coming from the server
this.hubConnection.on("Send", (message) => {
this.messages.push(message);
});
// starting the connection
this.hubConnection.start();
}
send() {
// message sent from the client to the server
this.hubConnection.invoke("Echo", this.message);
this.message = "";
}
}
Updating the HTML to bind to component
<input type="text" [(ngModel)]="message">
<button (click)="send()">Send</button>
<p *ngFor="let m of messages">{{m}}</p>
And that's it!!!
Hope it helps.
Cheers.